In this example, I’ll show you how to cut, copy, paste and clear text in the clipboard. Cutting and copying text is really straight forward, pasting text requires just a little bit of checking. For example, we want to check to see what the clipboard contains before we try and do something like pasting an image into a text box.
To copy text, its just a simple one-liner:
Clipboard.SetText(txtTextBox.SelectedText) |
To cut text, it’s almost as simple as copying it, but we need to remember to delete the data from the text box once it’s been copied:
Clipboard.SetText(txtTextBox.SelectedText) txtTextBox.SelectedText = "" |
To paste text there’s several things we have to worry about like I mentioned above. We first want to check what’s in the clipboard and verify that the contents of the clipboard can be pasted into whatever field/object we are trying to paste them into. In this example, I’m going to use the GetText method to make sure I only get the text from the clipboard and paste it into the text field. In the example below, I’ll get the data from the clipboard, then check to see if it is text before pasting it.
txtTextBox.SelectedText = Clipboard.GetText |
Clik here to view.
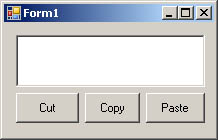
Cut Copy Paste for .NET Applications
This is the code for the above program written in VB.NET
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 | 'This code was developed by Gerardo Lopez and was downloaded from www.brangle.com 'Complete source code is available at: 'http://www.brangle.com/wordpress/2009/08/how-to-cutcopypaste-text-into-clipboard-using-vb-net/ Public Class Form1 Private Sub btnCut_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnCut.Click 'Checks to see if the user selected anything If txtTextBox.SelectedText <> "" Then 'Good, the user selected something 'Copy the information to the clipbaord Clipboard.SetText(txtTextBox.SelectedText) 'Since this is a cut command, we want to clear whatever 'text they had selected when they clicked cut txtTextBox.SelectedText = "" Else 'If there was no text selected, print out an error message box MsgBox("No text is selected to cut") End If End Sub Private Sub btnCopy_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnCopy.Click 'Checks to see if the user selected anything If txtTextBox.SelectedText <> "" Then 'Copy the information to the clipboard Clipboard.SetText(txtTextBox.SelectedText) Else 'If no text was selected, print out an error message box MsgBox("No text is selected to copy") End If End Sub Private Sub btnPaste_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnPaste.Click 'Get the data stored in the clipboard Dim iData As IDataObject = Clipboard.GetDataObject() 'Check to see if the data is in a text format If iData.GetDataPresent(DataFormats.Text) Then 'If it's text, then paste it into the textbox txtTextBox.SelectedText = CType(iData.GetData(DataFormats.Text), String) Else 'If it's not text, print a warning message MsgBox("Data in the clipboard is not availble for entry into a textbox") End If End Sub End Class |
Now in general, I don’t think that the clipboard should be cleared by the programmer, it should be cleared by the user. But if you have a legitimate reason to clear the clipboard, here’s the code to do just that:
Clipboard.Clear() |